Use the correct order on expected and actual parameters when making assertions
PHPUnit allows you to do basic assertions when testing your app. For our example we are going to use one basic, the assertSame assertion. This does a strict check on our values and passes when the 2 values are exactly the same, and they have the same type.
Let's see the definition of the function.
final public static function assertSame(mixed $expected, mixed $actual, string $message = ''): void
As you can see, we can pass $expected and $actual parameters.
You may ask if the order of $expected and $actual really matters, since it's just an equal check.
Both of the below examples should work
$this->assertSame('test@test.com', $user->email);
$this->assertSame($user->email, 'test@test.com');
Should be the same, right?
Well, they are the same on their functionality, but they are not the same when it comes to informing the user why this test may fails at times.
If you want to check that the newly created $user (with email test@test.com) has the correct email that you specified:
Using the correct order:
$this->assertSame('test1@test.com', $user->email);
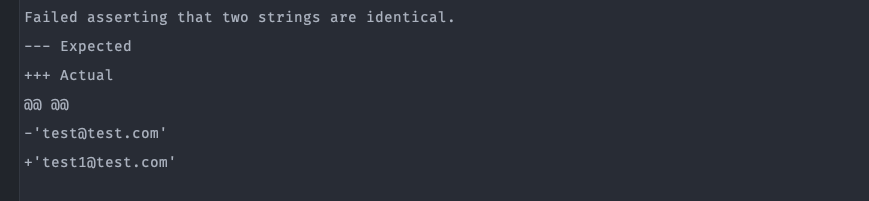
Using the wrong order:
$this->assertSame($user->email, 'test1@test.com');
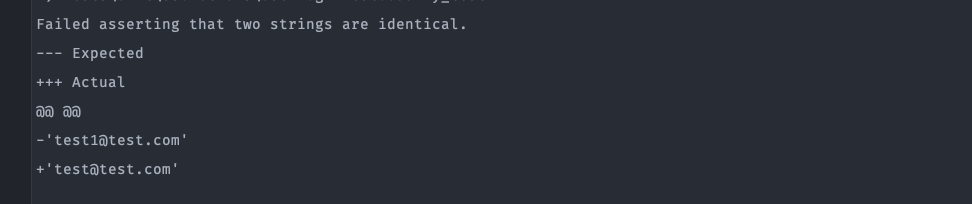
Basically the fail output is not what it meant to be, logically talking, when using the wrong order.
So it may confuses other developers when trying to debug this test.
So just follow the correct order, especially if you are working on a team. It's a small detail where you literally have nothing extra to do, just follow it and you are set!